datastructures
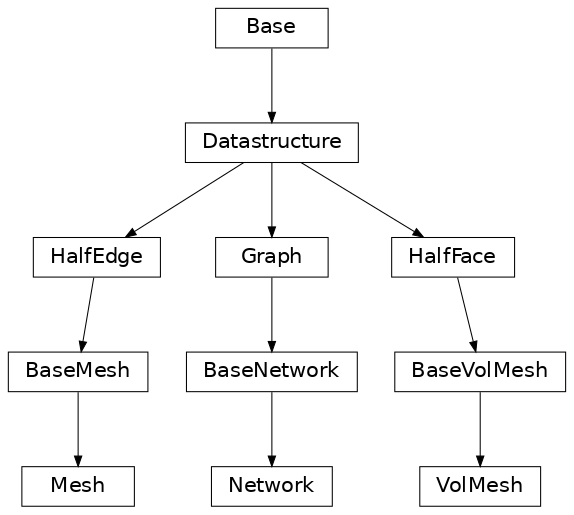
Meshes
Classes
Implementation of the base mesh data structure that adds some of the mesh algorithms as methods. |
Base Classes
Base half-edge data structure for representing meshes. |
|
Geometric implementation of a half edge data structure for polygon meshses. |
Functions
Compute the (axis aligned) bounding box of a mesh. |
|
Compute the (axis aligned) bounding box of a projection of the mesh in the XY plane. |
|
Compute the contours of the mesh. |
|
Generates the dual mesh from a seed mesh. |
|
Generates the join mesh from a seed mesh. |
|
Generates the ambo mesh from a seed mesh. |
|
Generates the kis mesh from a seed mesh. |
|
Generates the needle mesh from a seed mesh. |
|
Generates the zip mesh from a seed mesh. |
|
Generates the truncate mesh from a seed mesh. |
|
Generates the ortho mesh from a seed mesh. |
|
Generates the expand mesh from a seed mesh. |
|
Generates the gyro mesh from a seed mesh. |
|
Generates the snub mesh from a seed mesh. |
|
Generates the meta mesh from a seed mesh. |
|
Generates the bevel mesh from a seed mesh. |
|
Cull all duplicate vertices of a mesh and sanitize affected faces. |
|
Construct the dual of a mesh. |
|
Explode a mesh into its disconnected parts. |
|
Build a face adjacency dict. |
|
Flip the cycle directions of all faces. |
|
Compute geodesic from the vertices of a mesh to given source vertices. |
|
Verify that the mesh is connected. |
|
Compute the isolines of a specified attribute of the vertices of a mesh. |
|
Merge two faces of a mesh over their shared edge. |
|
Offset a mesh. |
|
Compute the (axis aligned) bounding box of a mesh. |
|
Compute the (axis aligned) bounding box of a projection of the mesh in the XY plane. |
|
Planarise a set of connected faces. |
|
Slice a mesh with a plane and construct the resulting submeshes. |
|
Smooth a mesh by moving every free vertex to the centroid of its neighbors. |
|
Smooth a mesh by moving each vertex to the barycenter of the centroids of the surrounding faces, weighted by area. |
|
Subdivide the input mesh. |
|
Subdivide a mesh using simple insertion of vertices. |
|
Subdivide a mesh by cutting corners. |
|
Subdivide a mesh such that all faces are quads. |
|
Subdivide a mesh using the Catmull-Clark algorithm. |
|
Subdivide a mesh following the doo-sabin scheme. |
|
Thicken a mesh. |
|
Transform a mesh. |
|
Transform a copy of |
|
Transform a mesh. |
|
Transform a copy of |
|
Unify the cycle directions of all faces. |
|
Weld vertices of a mesh within some precision distance. |
|
Join meshes without welding. |
|
Join and and weld meshes within some precision distance. |
|
Get data on circumcentre of triangular face. |
|
Compute the gaussian curvature at the vertices of a triangle mesh using the angular deficit. |
Matrices
Creates a vertex adjacency matrix from a Mesh datastructure. |
|
Creates a connectivity matrix from a Mesh datastructure. |
|
Creates a vertex degree matrix from a Mesh datastructure. |
|
Construct the face matrix from a Mesh datastructure. |
|
Construct a Laplacian matrix with uniform weights from a mesh data structure. |
Networks
Base Classes
Base graph data structure for describing the topological relationships between nodes connected by edges. |
|
Geometric implementation of a basic edge graph. |
Functions
Generate the complement network of a network. |
|
Count the number of crossings (pairs of crossing edges) in the network. |
|
Embed the network in the plane. |
|
Identify all pairs of crossing edges in a network. |
|
Find the faces of a network. |
|
Verify that the network is connected. |
|
Verify if a network has crossing edges. |
|
Check if the network is planar. |
|
Verify that a network is embedded in the plane without crossing edges. |
|
Verify that a network lies in the XY plane. |
|
Transform a network. |
|
Transform a copy of |