Surface JSON Data
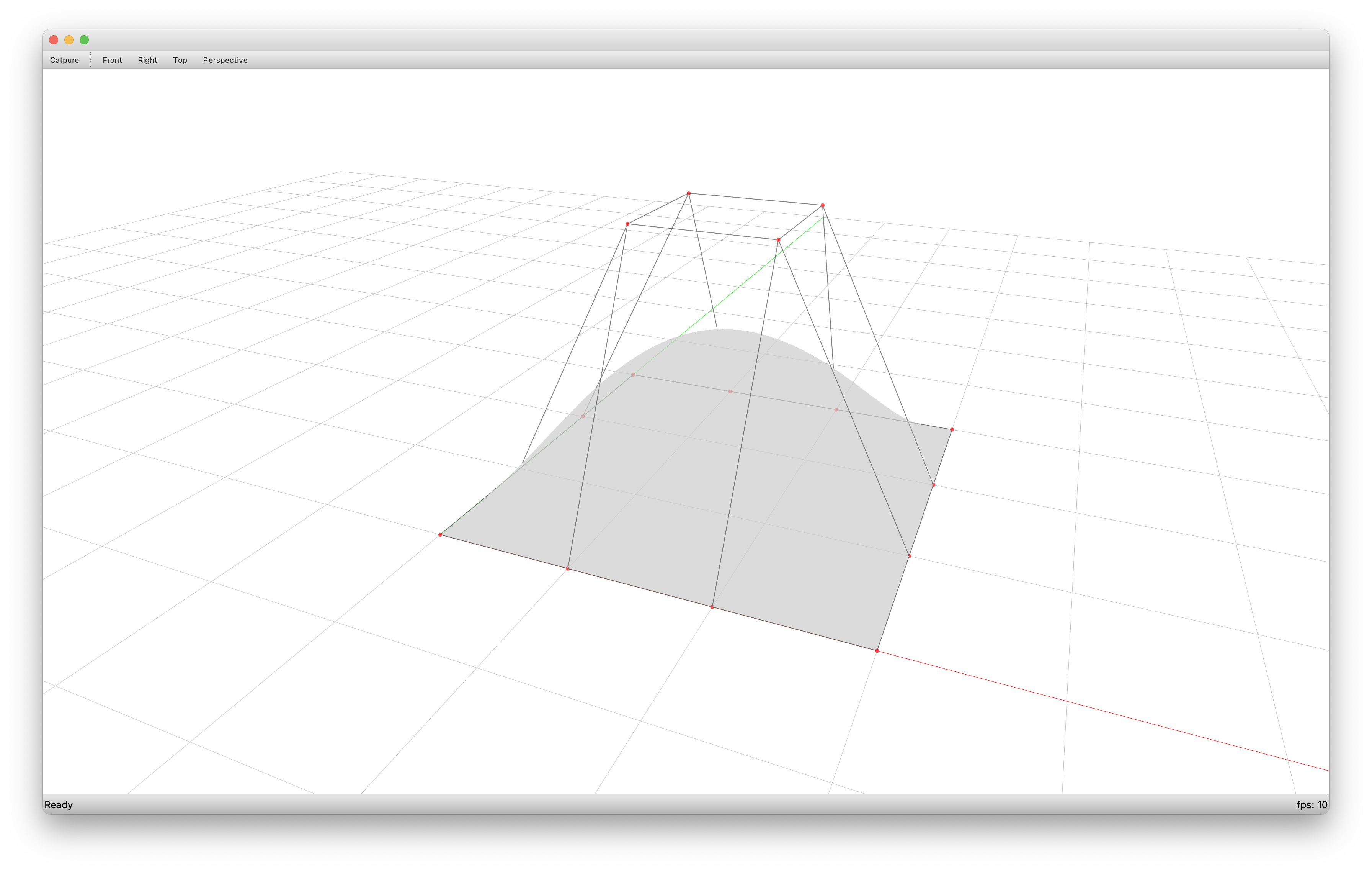
from compas_viewer import Viewer
from compas.colors import Color
from compas.geometry import NurbsSurface
from compas.geometry import Point
from compas.geometry import Polyline
points = [
[Point(0, 0, 0), Point(1, 0, 0), Point(2, 0, 0), Point(3, 0, 0)],
[Point(0, 1, 0), Point(1, 1, 2), Point(2, 1, 2), Point(3, 1, 0)],
[Point(0, 2, 0), Point(1, 2, 2), Point(2, 2, 2), Point(3, 2, 0)],
[Point(0, 3, 0), Point(1, 3, 0), Point(2, 3, 0), Point(3, 3, 0)],
]
surface = NurbsSurface.from_points(points=points)
# ==============================================================================
# JSON Data
# ==============================================================================
string = surface.to_jsonstring(pretty=True)
print(string)
other = NurbsSurface.from_jsonstring(string)
print(surface == other)
# ==============================================================================
# Visualisation
# ==============================================================================
viewer = Viewer()
viewer.scene.add(other) # type: ignore
# control polygon of original
points = list(surface.points)
viewer.scene.add([Polyline(row) for row in points], linewidth=1, linecolor=Color(0.3, 0.3, 0.3))
viewer.scene.add([Polyline(col) for col in zip(*points)], linewidth=1, linecolor=Color(0.3, 0.3, 0.3))
viewer.scene.add(points, pointsize=10)
# isocurves of original
u_curves = []
for u in surface.space_u(7): # type: ignore
u_curves.append(surface.isocurve_u(u).to_polyline())
v_curves = []
for v in surface.space_v(7): # type: ignore
v_curves.append(surface.isocurve_v(v).to_polyline())
viewer.scene.add(u_curves, linecolor=Color(0.8, 0.8, 0.8), linewidth=3)
viewer.scene.add(v_curves, linecolor=Color(0.8, 0.8, 0.8), linewidth=3)
viewer.show()
{
"is_u_periodic": false,
"is_v_periodic": false,
"points": [
[
[
0.0,
0.0,
0.0
],
[
1.0,
0.0,
0.0
],
[
2.0,
0.0,
0.0
],
[
3.0,
0.0,
0.0
]
],
[
[
0.0,
1.0,
0.0
],
[
1.0,
1.0,
2.0
],
[
2.0,
1.0,
2.0
],
[
3.0,
1.0,
0.0
]
],
[
[
0.0,
2.0,
0.0
],
[
1.0,
2.0,
2.0
],
[
2.0,
2.0,
2.0
],
[
3.0,
2.0,
0.0
]
],
[
[
0.0,
3.0,
0.0
],
[
1.0,
3.0,
0.0
],
[
2.0,
3.0,
0.0
],
[
3.0,
3.0,
0.0
]
]
],
"u_degree": 3,
"u_knots": [
0.0,
1.0
],
"u_mults": [
4,
4
],
"v_degree": 3,
"v_knots": [
0.0,
1.0
],
"v_mults": [
4,
4
],
"weights": [
[
1.0,
1.0,
1.0,
1.0
],
[
1.0,
1.0,
1.0,
1.0
],
[
1.0,
1.0,
1.0,
1.0
],
[
1.0,
1.0,
1.0,
1.0
]
]
}