Mesh Slicing
This example demonstrates how to slice a mesh with multiple planes using COMPAS CGAL.
Key Features:
Loading STL mesh files
Creating multiple slice planes from bounding box
Slicing mesh with multiple planes
Converting slice results to polylines
Visualization with semi-transparent mesh and slice curves
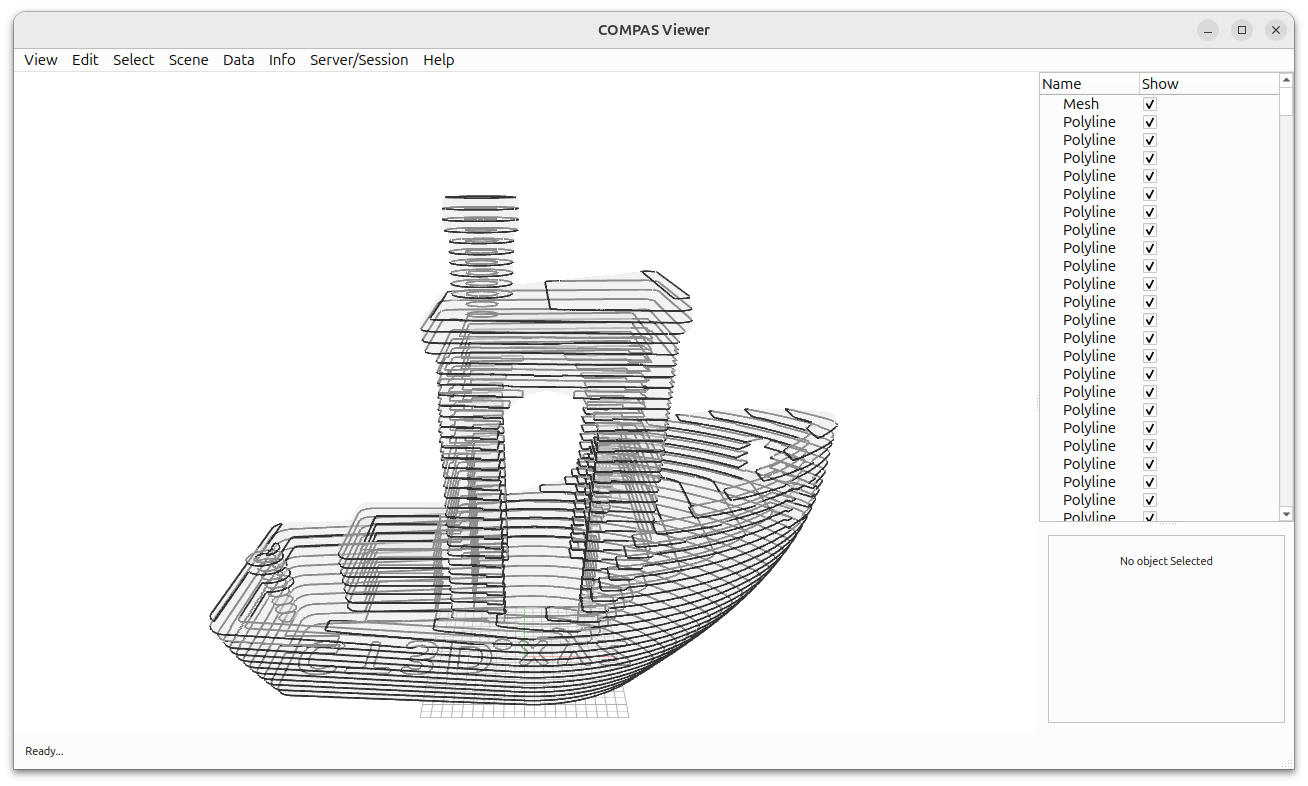
import math
from pathlib import Path
import numpy as np
from compas.datastructures import Mesh
from compas.geometry import Plane
from compas.geometry import Point
from compas.geometry import Polyline
from compas.geometry import Vector
from compas_viewer import Viewer
from compas_viewer.config import Config
from line_profiler import profile
from compas_cgal.slicer import slice_mesh
@profile
def main():
# Get Mesh from STL
FILE = Path(__file__).parent.parent.parent / "data" / "3DBenchy.stl"
benchy = Mesh.from_stl(FILE)
V, F = benchy.to_vertices_and_faces()
# Get Slice planes from the bounding box
bbox = benchy.aabb()
normal = Vector(0, 0, 1)
planes = []
for i in np.linspace(bbox.zmin, bbox.zmax, 50):
plane = Plane(Point(0, 0, i), normal)
planes.append(plane)
# Slice
slicer_polylines = slice_mesh((V, F), planes)
# Convert edges to polylines
polylines = []
for polyline in slicer_polylines:
points = []
for point in polyline:
points.append(Point(*point))
polylines.append(Polyline(points))
return benchy, polylines
mesh, polylines = main()
# ==============================================================================
# Visualize
# ==============================================================================
config = Config()
config.camera.target = [0, 100, 0]
config.camera.position = [0, -75, 50]
config.camera.scale = 10
viewer = Viewer(config=config)
viewer.scene.add(mesh, opacity=0.5, show_lines=False, show_points=False)
for polyline in polylines:
viewer.scene.add(polyline, linewidth=2, show_points=False)
viewer.show()